0134-加油站
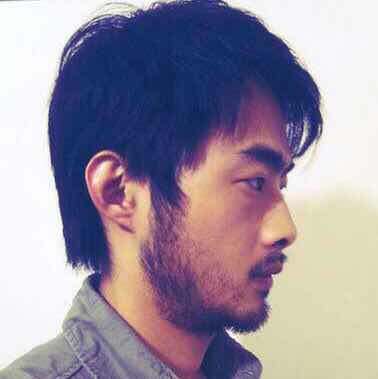
在一条环路上有 n
个加油站,其中第 i
个加油站有汽油 gas[i]
_ _ 升。
你有一辆油箱容量无限的的汽车,从第 __i
__ 个加油站开往第 __i+1
_ _ 个加油站需要消耗汽油 cost[i]
_ _
升。你从其中的一个加油站出发,开始时油箱为空。
给定两个整数数组 gas
和 cost
,如果你可以按顺序绕环路行驶一周,则返回出发时加油站的编号,否则返回 -1
。如果存在解,则
保证 它是 唯一 的。
示例 1:
**输入:** gas = [1,2,3,4,5], cost = [3,4,5,1,2]
**输出:** 3
**解释:** 从 3 号加油站(索引为 3 处)出发,可获得 4 升汽油。此时油箱有 = 0 + 4 = 4 升汽油
开往 4 号加油站,此时油箱有 4 - 1 + 5 = 8 升汽油
开往 0 号加油站,此时油箱有 8 - 2 + 1 = 7 升汽油
开往 1 号加油站,此时油箱有 7 - 3 + 2 = 6 升汽油
开往 2 号加油站,此时油箱有 6 - 4 + 3 = 5 升汽油
开往 3 号加油站,你需要消耗 5 升汽油,正好足够你返回到 3 号加油站。
因此,3 可为起始索引。
示例 2:
**输入:** gas = [2,3,4], cost = [3,4,3]
**输出:** -1
**解释:** 你不能从 0 号或 1 号加油站出发,因为没有足够的汽油可以让你行驶到下一个加油站。
我们从 2 号加油站出发,可以获得 4 升汽油。 此时油箱有 = 0 + 4 = 4 升汽油
开往 0 号加油站,此时油箱有 4 - 3 + 2 = 3 升汽油
开往 1 号加油站,此时油箱有 3 - 3 + 3 = 3 升汽油
你无法返回 2 号加油站,因为返程需要消耗 4 升汽油,但是你的油箱只有 3 升汽油。
因此,无论怎样,你都不可能绕环路行驶一周。
提示:
gas.length == n
cost.length == n
1 <= n <= 105
0 <= gas[i], cost[i] <= 104
方法一:一次遍历
思路与算法
最容易想到的解法是:从头到尾遍历每个加油站,并检查以该加油站为起点,最终能否行驶一周。我们可以通过减小被检查的加油站数目,来降低总的时间复杂度。
假设我们此前发现,从加油站 $x$ 出发,每经过一个加油站就加一次油(包括起始加油站),最后一个可以到达的加油站是 $y$(不妨设 $x<y$)。这就说明:
$$
\sum_{i=x}^{y}\textit{gas}[i] < \sum_{i=x}^{y}\textit{cost}[i] \
\sum_{i=x}^{j}gas[i] \ge \sum_{i=x}^{j}cost[i] ~ \text{(For all $j \in [x, y)$) }
$$
第一个式子表明无法到达加油站 $y$ 的下一个加油站,第二个式子表明可以到达 $y$ 以及 $y$ 之前的所有加油站。
现在,考虑任意一个位于 $x,y$ 之间的加油站 $z$(包括 $x$ 和 $y$),我们现在考察从该加油站出发,能否到达加油站 $y$ 的下一个加油站,也就是要判断 $\sum_{i=z}^{y}\textit{gas}[i]$ 与 $\sum_{i=z}^{y}\textit{cost}[i]$ 之间的大小关系。
根据上面的式子,我们得到:
$$
\begin{aligned}
\sum_{i=z}^{y}\textit{gas}[i]&=\sum_{i=x}^{y}\textit{gas}[i]-\sum_{i=x}^{z-1}\textit{gas}[i] \
&< \sum_{i=x}^{y}\textit{cost}[i]-\sum_{i=x}^{z-1}\textit{gas}[i] \
&< \sum_{i=x}^{y}\textit{cost}[i]-\sum_{i=x}^{z-1}cost[i] \
&= \sum_{i=z}^{y}\textit{cost}[i]
\end{aligned}
$$
其中不等式的第二步、第三步分别利用了上面的第一个、第二个不等式。
从上面的推导中,能够得出结论:从 $x,y$ 之间的任何一个加油站出发,都无法到达加油站 $y$ 的下一个加油站。
在发现了这一个性质后,算法就很清楚了:我们首先检查第 $0$ 个加油站,并试图判断能否环绕一周;如果不能,就从第一个无法到达的加油站开始继续检查。
代码
1 | class Solution { |
1 | class Solution { |
1 | func canCompleteCircuit(gas []int, cost []int) int { |
1 | int canCompleteCircuit(int* gas, int gasSize, int* cost, int costSize) { |
1 | var canCompleteCircuit = function(gas, cost) { |
复杂度分析
时间复杂度:$O(N)$,其中 $N$ 为数组的长度。我们对数组进行了单次遍历。
空间复杂度:$O(1)$。