0492-构造矩形
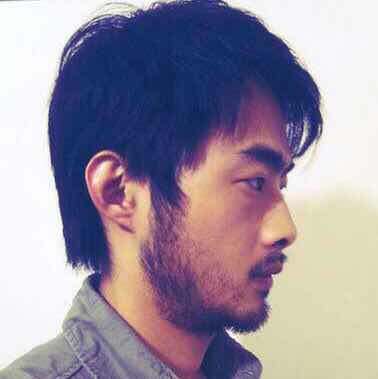
作为一位web开发者, 懂得怎样去规划一个页面的尺寸是很重要的。 所以,现给定一个具体的矩形页面面积,你的任务是设计一个长度为 L 和宽度为 W
且满足以下要求的矩形的页面。要求:
- 你设计的矩形页面必须等于给定的目标面积。
- 宽度
W
不应大于长度L
,换言之,要求L >= W
。 - 长度
L
和宽度W
之间的差距应当尽可能小。
返回一个 数组 [L, W]
,其中 _L
和 W
是你按照顺序设计的网页的长度和宽度_。
示例1:
**输入:** 4
**输出:** [2, 2]
**解释:** 目标面积是 4, 所有可能的构造方案有 [1,4], [2,2], [4,1]。
但是根据要求2,[1,4] 不符合要求; 根据要求3,[2,2] 比 [4,1] 更能符合要求. 所以输出长度 L 为 2, 宽度 W 为 2。
示例 2:
**输入:** area = 37
**输出:** [37,1]
示例 3:
**输入:** area = 122122
**输出:** [427,286]
提示:
1 <= area <= 107
方法一:数学
根据题目给出的三个要求,可知:
- L\cdot W=\textit{area,这也意味着 area 可以被 W 整除;
- L\ge W,结合要求 1 可得 W\cdot W\le L\cdot W=\textit{area,从而有 W\le\lfloor\sqrt\textit{area}\rfloor;
- 这意味着 W 应取满足 area 可以被 W 整除且 W\le\lfloor\sqrt\textit{area}\rfloor 的最大值。
我们可以初始化 W=\lfloor\sqrt\textit{area}\rfloor,不断循环判断 area 能否被 W 整除,如果可以则跳出循环,否则将 W 减一后继续循环。
循环结束后我们就找到了答案,长为 \dfrac{\textit{area}}{W,宽为 W。
1 | class Solution: |
1 | class Solution { |
1 | class Solution { |
1 | public class Solution { |
1 | func constructRectangle(area int) []int { |
1 | var constructRectangle = function(area) { |
复杂度分析
时间复杂度:O(\sqrt\textit{area})。当 area 为质数时为最坏情况。
空间复杂度:O(1)。
Comments