0827-最大人工岛
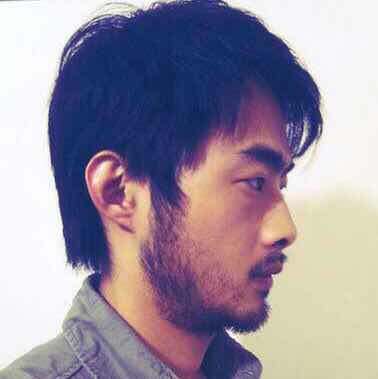
给你一个大小为 n x n
二进制矩阵 grid
。 最多 只能将一格 0
变成 1
。
返回执行此操作后,grid
中最大的岛屿面积是多少?
岛屿 由一组上、下、左、右四个方向相连的 1
形成。
示例 1:
**输入:** grid = [[1, 0], [0, 1]]
**输出:** 3
**解释:** 将一格0变成1,最终连通两个小岛得到面积为 3 的岛屿。
示例 2:
**输入:** grid = **** [[1, 1], [1, 0]]
**输出:** 4
**解释:** 将一格0变成1,岛屿的面积扩大为 4。
示例 3:
**输入:** grid = [[1, 1], [1, 1]]
**输出:** 4
**解释:** 没有0可以让我们变成1,面积依然为 4。
提示:
n == grid.length
n == grid[i].length
1 <= n <= 500
grid[i][j]
为0
或1
方法一:标记岛屿 + 合并
我们给每个岛屿进行标记,标记值与岛屿的某个 grid}[i][j] 有关,即 t = i \times n + j + 1,t 唯一。使用 tag 记录每个点所属的岛屿的标记,并且使用哈希表 area 保存每个岛屿的面积。岛屿的面积可以使用深度优先搜索或广度优先搜索计算。
对于每个 grid}[i][j] = 0,我们计算将它变为 1 后,新合并的岛屿的面积 z(z 的初始值为 1,对应该点的面积):使用集合 connected 保存与 grid}[i][j] 相连的岛屿,遍历与 grid}[i][j] 相邻的四个点,如果该点的值为 1,且它所在的岛屿并不在集合中,我们将 z 加上该点所在的岛屿面积,并且将该岛屿加入集合中。所有这些新合并岛屿以及原来的岛屿的面积的最大值就是最大的岛屿面积。
1 | class Solution: |
1 | const vector<int> d = {0, -1, 0, 1, 0}; |
1 | class Solution { |
1 | public class Solution { |
1 |
|
1 | const d = [0, -1, 0, 1, 0]; |
1 | func largestIsland(grid [][]int) (ans int) { |
复杂度分析
时间复杂度:O(n^2),其中 n 是 grid 的长与宽。使用深度优先搜索获取岛屿面积时,总共访问不超过 n^2 个点。
空间复杂度:O(n^2)。保存 tag 与 area 需要 O(n^2) 的空间。
Comments