0957-N 天后的牢房
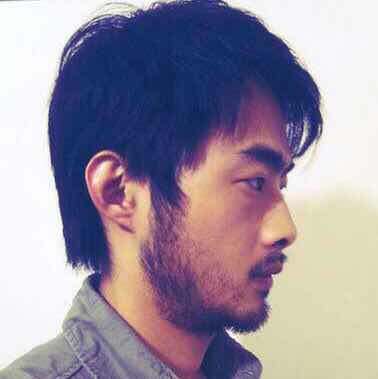
监狱中 8
间牢房排成一排,每间牢房可能被占用或空置。
每天,无论牢房是被占用或空置,都会根据以下规则进行变更:
- 如果一间牢房的两个相邻的房间都被占用或都是空的,那么该牢房就会被占用。
- 否则,它就会被空置。
注意 :由于监狱中的牢房排成一行,所以行中的第一个和最后一个牢房不存在两个相邻的房间。
给你一个整数数组 cells
,用于表示牢房的初始状态:如果第 i
间牢房被占用,则 cell[i]==1
,否则 cell[i]==0
。另给你一个整数 n
。
请你返回 n
天后监狱的状况(即,按上文描述进行 n
次变更)。
示例 1:
**输入:** cells = [0,1,0,1,1,0,0,1], n = 7
**输出:** [0,0,1,1,0,0,0,0]
**解释:** 下表总结了监狱每天的状况:
Day 0: [0, 1, 0, 1, 1, 0, 0, 1]
Day 1: [0, 1, 1, 0, 0, 0, 0, 0]
Day 2: [0, 0, 0, 0, 1, 1, 1, 0]
Day 3: [0, 1, 1, 0, 0, 1, 0, 0]
Day 4: [0, 0, 0, 0, 0, 1, 0, 0]
Day 5: [0, 1, 1, 1, 0, 1, 0, 0]
Day 6: [0, 0, 1, 0, 1, 1, 0, 0]
Day 7: [0, 0, 1, 1, 0, 0, 0, 0]
示例 2:
**输入:** cells = [1,0,0,1,0,0,1,0], n = 1000000000
**输出:** [0,0,1,1,1,1,1,0]
提示:
cells.length == 8
cells[i]
为0
或1
1 <= n <= 109
方法 1:模拟
想法
模拟每一天监狱的状态。
由于监狱最多只有 256 种可能的状态,所以状态一定会快速的形成一个循环。我们可以当状态循环出现的时候记录下循环的周期 t
然后跳过 t
的倍数的天数。
算法
实现一个简单的模拟,每次迭代一天的情况。对于每一天,我们减少剩余的天数 N
,然后将监狱状态改变成(state -> nextDay(state)
)。
如果我们到达一个已经访问的状态,并且知道距当前过去了多久,设为 t
,那么由于这是一个循环,可以让 N %= t
。这确保了我们的算法只需要执行 O(2^{\text{cells.length}}) 步。
1 | class Solution { |
1 | class Solution(object): |
复杂度分析
- 时间复杂度:O(2^N),其中 N 是监狱房间的个数。
- 空间复杂度:O(2^N * N)。
Comments