0972-相等的有理数
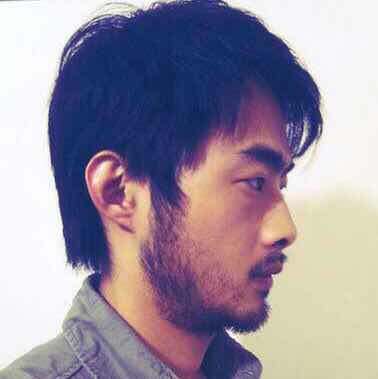
给定两个字符串 s
和 t
,每个字符串代表一个非负有理数,只有当它们表示相同的数字时才返回 true
。字符串中可以使用括号来表示有理数的重复部分。
有理数 最多可以用三个部分来表示: 整数部分 <IntegerPart>
、 小数非重复部分<NonRepeatingPart>
和 小数重复部分 <(><RepeatingPart><)>
。数字可以用以下三种方法之一来表示:
<IntegerPart>
- 例:
0
,12
和123
- 例:
<IntegerPart><.><NonRepeatingPart>
- 例:
0.5 , ``1.
,2.12
和123.0001
- 例:
<IntegerPart><.><NonRepeatingPart><(><RepeatingPart><)>
- 例:
0.1(6)
,1.(9)
,123.00(1212)
- 例:
十进制展开的重复部分通常在一对圆括号内表示。例如:
1 / 6 = 0.16666666... = 0.1(6) = 0.1666(6) = 0.166(66)
示例 1:
**输入:** s = "0.(52)", t = "0.5(25)"
**输出:** true
**解释:** 因为 "0.(52)" 代表 0.52525252...,而 "0.5(25)" 代表 0.52525252525.....,则这两个字符串表示相同的数字。
示例 2:
**输入:** s = "0.1666(6)", t = "0.166(66)"
**输出:** true
示例 3:
**输入:** s = "0.9(9)", t = "1."
**输出:** true
**解释:** "0.9(9)" 代表 0.999999999... 永远重复,等于 1 。[[有关说明,请参阅此链接](https://baike.baidu.com/item/0.999…/5615429?fr=aladdin)]
"1." 表示数字 1,其格式正确:(IntegerPart) = "1" 且 (NonRepeatingPart) = "" 。
提示:
- 每个部分仅由数字组成。
- 整数部分
<IntegerPart>
不会以零开头。(零本身除外) 1 <= <IntegerPart>.length <= 4
0 <= <NonRepeatingPart>.length <= 4
1 <= <RepeatingPart>.length <= 4
方法:分数类
思路
因为给定的两个数字都表示一个分数,所以我们需要一个分数类去处理这两个分数。它应该能帮助我们将两个分数加起来,并且保证答案为最简形式。
算法
我们需要理解给定的两个分数,最困难的问题是如何表示它们。
比如说我们有一个字符串 S = "0.(12)"
。它代表(定义 r = 1/100):
S = 12/100} + 12/10000} + 12/10^6} + 12/10^8} + 12/10^{10}} + \cdots
S = 12 * (r + r^2 + r^3 + \cdots)
S = 12 * r}{1-r}
其中 (r + r^2 + r^3 + \cdots) 是一个等比数列求和问题。
总而言之,对于长度为 k 的重复部分 x,会对答案有 xr}{1-r 的贡献,其中 r = 10^{-k。
另外两部分就更容易计算了,因为它们仅仅是对数值的简单翻译。
1 | class Solution { |
1 | from fractions import Fraction |
复杂度分析
时间复杂度:O(1),因为字符串 S, T 的长度可以看作是 O(1) 级别的。
空间复杂度:O(1)。
Comments