1061-按字典序排列最小的等效字符串
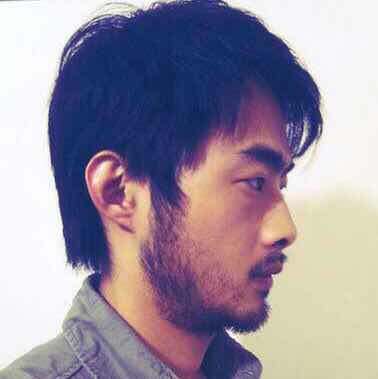
给出长度相同的两个字符串s1
和 s2
,还有一个字符串 baseStr
。
其中 s1[i]
和 s2[i]
是一组等价字符。
- 举个例子,如果
s1 = "abc"
且s2 = "cde"
,那么就有'a' == 'c', 'b' == 'd', 'c' == 'e'
。
等价字符遵循任何等价关系的一般规则:
- ** 自反性 **:
'a' == 'a'
- **对称性 **:
'a' == 'b'
则必定有'b' == 'a'
- 传递性 :
'a' == 'b'
且'b' == 'c'
就表明'a' == 'c'
例如, s1 = "abc"
和 s2 = "cde"
的等价信息和之前的例子一样,那么 baseStr = "eed"
, "acd"
或"aab"
,这三个字符串都是等价的,而 "aab"
是 baseStr
的按字典序最小的等价字符串
利用 _ _s1
和 s2
的等价信息,找出并返回 _ _baseStr
_ _ 的按字典序排列最小的等价字符串。
示例 1:
**输入:** s1 = "parker", s2 = "morris", baseStr = "parser"
**输出:** "makkek"
**解释:** 根据 A 和 B 中的等价信息,我们可以将这些字符分为 [m,p], [a,o], [k,r,s], [e,i] 共 4 组。每组中的字符都是等价的,并按字典序排列。所以答案是 "makkek"。
示例 2:
**输入:** s1 = "hello", s2 = "world", baseStr = "hold"
**输出:** "hdld"
**解释:** 根据 A 和 B 中的等价信息,我们可以将这些字符分为 [h,w], [d,e,o], [l,r] 共 3 组。所以只有 S 中的第二个字符 'o' 变成 'd',最后答案为 "hdld"。
示例 3:
**输入:** s1 = "leetcode", s2 = "programs", baseStr = "sourcecode"
**输出:** "aauaaaaada"
**解释:** 我们可以把 A 和 B 中的等价字符分为 [a,o,e,r,s,c], [l,p], [g,t] 和 [d,m] 共 4 组,因此 S 中除了 'u' 和 'd' 之外的所有字母都转化成了 'a',最后答案为 "aauaaaaada"。
提示:
1 <= s1.length, s2.length, baseStr <= 1000
s1.length == s2.length
- 字符串
s1
,s2
, andbaseStr
仅由从'a'
到'z'
的小写英文字母组成。
解题思路
本题是典型的并查集应用题,一个字符可以与多个字符等价,很容易联想到并查集的union,本题我们将‘a’-“z”26个字符,可以转化为0-25这26个数字。
由于题目要求:将baseStr转化为字典序最小的等价字符串;那么我们需要在union方法中做一个改造,使得等价的两个字符union时,根节点指向字典序更小的那个字符。
1 | if (pRoot < qRoot) { |
解题步骤:
- 改造UF的union方法,使得等价的两个字符union时,根节点指向字典序更小的那个字符;
- 同时遍历s1、s2,将等价的字符相连;
- 遍历baseStr,找到每个字符的根,并用sb组合;
- 返回结果。
代码
1 | class Solution { |
如果您认可道哥的题解,麻烦点赞支持一下。
Comments