给你一个字符串 text
,你需要使用 text
中的字母来拼凑尽可能多的单词 “ balloon”(气球)。
字符串 text
中的每个字母最多只能被使用一次。请你返回最多可以拼凑出多少个单词 **” balloon”**。
示例 1:
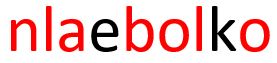
**输入:** text = "nlaebolko"
**输出:** 1
示例 2:

**输入:** text = "loonbalxballpoon"
**输出:** 2
示例 3:
**输入:** text = "leetcode"
**输出:** 0
提示:
1 <= text.length <= 10^4
text
全部由小写英文字母组成
方法一:统计
思路
构成单词 “balloon” 需要 1 个字母 b' 、1 个字母
a’ 、2 个字母 l' 、2 个字母
o’ 、1 个字母 n',因此只需要统计字符串中字母
a’,b',
l’,o',
n’ 的数量即可。其中每个字母 “balloon” 需要两个 l',
o’,可以将字母 l',
o’ 的数量除以 2,返回字母 a',
b’,l',
o’,`n’ 中数量最小值即为可以构成的单词数量。
代码
[sol1-Python3]1 2 3 4 5 6
| class Solution: def maxNumberOfBalloons(self, text: str) -> int: cnt = Counter(ch for ch in text if ch in "balon") cnt['l'] //= 2 cnt['o'] //= 2 return min(cnt.values()) if len(cnt) == 5 else 0
|
[sol1-C++]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| class Solution { public: int maxNumberOfBalloons(string text) { vector<int> cnt(5); for (auto & ch : text) { if (ch == 'b') { cnt[0]++; } else if (ch == 'a') { cnt[1]++; } else if (ch == 'l') { cnt[2]++; } else if (ch == 'o') { cnt[3]++; } else if (ch == 'n') { cnt[4]++; } } cnt[2] /= 2; cnt[3] /= 2; return *min_element(cnt.begin(), cnt.end()); } };
|
[sol1-Java]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| class Solution { public int maxNumberOfBalloons(String text) { int[] cnt = new int[5]; for (int i = 0; i < text.length(); ++i) { char ch = text.charAt(i); if (ch == 'b') { cnt[0]++; } else if (ch == 'a') { cnt[1]++; } else if (ch == 'l') { cnt[2]++; } else if (ch == 'o') { cnt[3]++; } else if (ch == 'n') { cnt[4]++; } } cnt[2] /= 2; cnt[3] /= 2; return Arrays.stream(cnt).min().getAsInt(); } }
|
[sol1-C#]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class Solution { public int MaxNumberOfBalloons(string text) { int[] cnt = new int[5]; foreach (char ch in text) { if (ch == 'b') { cnt[0]++; } else if (ch == 'a') { cnt[1]++; } else if (ch == 'l') { cnt[2]++; } else if (ch == 'o') { cnt[3]++; } else if (ch == 'n') { cnt[4]++; } } cnt[2] /= 2; cnt[3] /= 2; return cnt.Min(); } }
|
[sol1-C]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| #define MIN(a, b) ((a) < (b) ? (a) : (b))
int maxNumberOfBalloons(char * text) { int cnt[5]; int n = strlen(text); memset(cnt, 0, sizeof(int) * 5); for (int i = 0; i < n; i++) { if (text[i] == 'b') { cnt[0]++; } else if (text[i] == 'a') { cnt[1]++; } else if (text[i] == 'l') { cnt[2]++; } else if (text[i] == 'o') { cnt[3]++; } else if (text[i] == 'n') { cnt[4]++; } } cnt[2] /= 2; cnt[3] /= 2; int res = INT_MAX; for (int i = 0; i < 5; i++) { res = MIN(res, cnt[i]); } return res; }
|
[sol1-Golang]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| func maxNumberOfBalloons(text string) int { cnt := [5]int{} for _, ch := range text { if ch == 'b' { cnt[0]++ } else if ch == 'a' { cnt[1]++ } else if ch == 'l' { cnt[2]++ } else if ch == 'o' { cnt[3]++ } else if ch == 'n' { cnt[4]++ } } cnt[2] /= 2 cnt[3] /= 2 ans := cnt[0] for _, v := range cnt[1:] { if v < ans { ans = v } } return ans }
|
[sol1-JavaScript]1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| var maxNumberOfBalloons = function(text) { const cnt = new Array(5).fill(0); for (const ch of text) { if (ch === 'b') { cnt[0]++; } else if (ch === 'a') { cnt[1]++; } else if (ch === 'l') { cnt[2]++; } else if (ch === 'o') { cnt[3]++; } else if (ch === 'n') { cnt[4]++; } } cnt[2] = Math.floor(cnt[2] / 2); cnt[3] = Math.floor(cnt[3] / 2); return _.min(cnt); };
|
复杂度分析