1507-转变日期格式
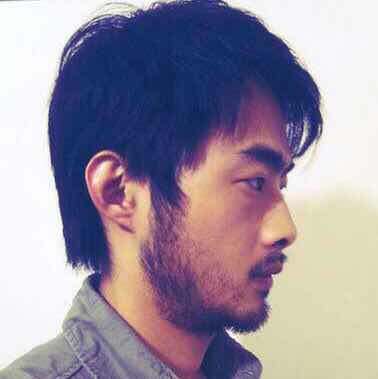
给你一个字符串 date
,它的格式为 Day Month Year
,其中:
Day
是集合{"1st", "2nd", "3rd", "4th", ..., "30th", "31st"}
中的一个元素。Month
是集合{"Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"}
中的一个元素。Year
的范围在 [1900, 2100]
之间。
请你将字符串转变为 YYYY-MM-DD
的格式,其中:
YYYY
表示 4 位的年份。MM
表示 2 位的月份。DD
表示 2 位的天数。
示例 1:
**输入:** date = "20th Oct 2052"
**输出:** "2052-10-20"
示例 2:
**输入:** date = "6th Jun 1933"
**输出:** "1933-06-06"
示例 3:
**输入:** date = "26th May 1960"
**输出:** "1960-05-26"
提示:
- 给定日期保证是合法的,所以不需要处理异常输入。
方法一:模拟
思路与算法
首先,我们可以按照空格把字符串分割成三部分,分别取出日、月、年。对于他们分别做这样的事情:
- 日:去掉结尾的两位英文字母,如果数字只有一位再补上前导零
- 月:使用字典映射的方式把月份的英文缩写转换成对应的数字
- 年:不用变化
最终组织成「年-月-日」的形式即可。
代码如下。
代码
1 | class Solution: |
1 | class Solution { |
1 | class Solution { |
复杂度分析
- 时间复杂度:O(1)。
- 空间复杂度:O(1)。
Comments