2185-统计包含给定前缀的字符串
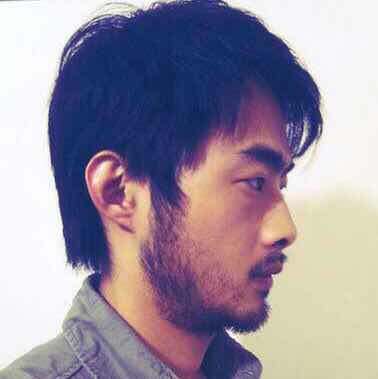
给你一个字符串数组 words
和一个字符串 pref
。
返回 words
__ 中以 pref
作为 前缀 的字符串的数目。
字符串 s
的 前缀 就是 s
的任一前导连续字符串。
示例 1:
**输入:** words = ["pay"," _ **at**_ tention","practice"," _ **at**_ tend"], pref = "at"
**输出:** 2
**解释:** 以 "at" 作为前缀的字符串有两个,分别是:" _ **at**_ tention" 和 " _ **at**_ tend" 。
示例 2:
**输入:** words = ["leetcode","win","loops","success"], pref = "code"
**输出:** 0
**解释:** 不存在以 "code" 作为前缀的字符串。
提示:
1 <= words.length <= 100
1 <= words[i].length, pref.length <= 100
words[i]
和pref
由小写英文字母组成
方法一:模拟
思路
按照题意,对 words 每个单词进行判断,是否以 pref 开头即可。最后返回满足条件的单词的数量。
代码
1 | class Solution: |
1 | class Solution { |
1 | public class Solution { |
1 | class Solution { |
1 | int prefixCount(char ** words, int wordsSize, char * pref) { |
1 | func prefixCount(words []string, pref string) (ans int) { |
1 | var prefixCount = function(words, pref) { |
复杂度分析
时间复杂度:O(n \times m),其中 n 是输入 words 的长度,m 是输入 pref 的长度。
空间复杂度:O(1),仅需要常数空间。
Comments