2239-找到最接近 0 的数字
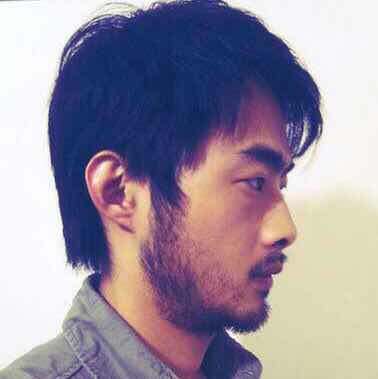
给你一个长度为 n
的整数数组 nums
,请你返回 nums
中最 接近 0
的数字。如果有多个答案,请你返回它们中的
最大值 。
示例 1:
**输入:** nums = [-4,-2,1,4,8]
**输出:** 1
**解释:**
-4 到 0 的距离为 |-4| = 4 。
-2 到 0 的距离为 |-2| = 2 。
1 到 0 的距离为 |1| = 1 。
4 到 0 的距离为 |4| = 4 。
8 到 0 的距离为 |8| = 8 。
所以,数组中距离 0 最近的数字为 1 。
示例 2:
**输入:** nums = [2,-1,1]
**输出:** 1
**解释:** 1 和 -1 都是距离 0 最近的数字,所以返回较大值 1 。
提示:
1 <= n <= 1000
-105 <= nums[i] <= 105
方法一:遍历
思路与算法
一个数与 0 的距离即为该数的绝对值,因此我们需要找出数组 nums 里面绝对值最小的元素的最大值。
我们遍历数组,并用 res 来维护已遍历元素中绝对值最小且数值最大的元素,以及 dis 来维护已遍历元素的最小绝对值。这两个变量的初值即为数组第一个元素的数值与绝对值。
当我们遍历到新的元素 num 时,我们需要比较该数绝对值 |\textit{num}| 与 dis 的关系,此时会有三种情况:
|\textit{num}| < \textit{dis,此时我们需要将 res 更新为 num,并将 dis 更新为 |\textit{num}|;
|\textit{num}| = \textit{dis,此时我们需要将 res 更新为 res 与 num 的最大值;
|\textit{num}| > \textit{dis,此时无需进行任何操作。
最终,res 即为数组 nums 里面绝对值最小的元素的最大值,我们返回该值作为答案。
代码
1 | class Solution { |
1 | class Solution: |
复杂度分析
时间复杂度:O(n),其中 n 为 nums 的长度。即为遍历寻找对应数字的时间复杂度。
空间复杂度:O(1)。
Comments