2423-删除字符使频率相同
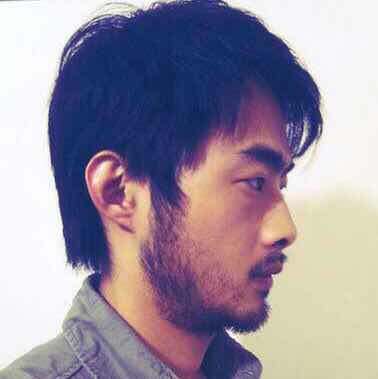
给你一个下标从 0 开始的字符串 word
,字符串只包含小写英文字母。你需要选择 一个 下标并 删除 下标处的字符,使得word
中剩余每个字母出现 频率 相同。
如果删除一个字母后,word
中剩余所有字母的出现频率都相同,那么返回 true
,否则返回 false
。
注意:
- 字母
x
的 频率 ** ** 是这个字母在字符串中出现的次数。 - 你 必须 恰好删除一个字母,不能一个字母都不删除。
示例 1:
**输入:** word = "abcc"
**输出:** true
**解释:** 选择下标 3 并删除该字母,word 变成 "abc" 且每个字母出现频率都为 1 。
示例 2:
**输入:** word = "aazz"
**输出:** false
**解释:** 我们必须删除一个字母,所以要么 "a" 的频率变为 1 且 "z" 的频率为 2 ,要么两个字母频率反过来。所以不可能让剩余所有字母出现频率相同。
提示:
2 <= word.length <= 100
word
只包含小写英文字母。
方法一:枚举
思路与算法
题目要求选择一个下标并删除下标处的字符,使得 word 中剩余每个字母出现频率相同。
暴力方法可以尝试删除不同下标处字符,复杂度为 O(n^2),其中 n 是字符串 word 的长度。
注意到删除不同位置的相同字符,不会改变剩余字符的频率,我们可以进行优化,只枚举删除不同的字符即可。
首先遍历输入字符串 word,统计每一个字符出现的频率。然后我们按照字母序,遍历所有的字符。如果当前这个字符出现在原字符串中,我们假定要删除这个字符,把这个字符出现的频率减一,统计所有出现字符的频率集合。如果集合大小为 1,则说明剩余每个字母出现频率相同,我们直接返回 true,反之说明删除当前字符不可行,我们把这个字符的频率加一进行还原。
最后,当我们尝试过所有不同字符后,还没有找到能删除的字符,使得满足要求,我们返回 false。
代码
1 | class Solution { |
1 | class Solution { |
1 | class Solution: |
1 | func equalFrequency(word string) bool { |
1 | public class Solution { |
1 | var equalFrequency = function(word) { |
1 | typedef struct { |
复杂度分析
时间复杂度:O(n+|\Sigma|^2),其中 n 是字符串 word 的长度, \Sigma 为字符集,在本题中字符集为所有小写字母,|\Sigma| = 26。
空间复杂度:O(|\Sigma|),\Sigma 为字符集,在本题中字符集为所有小写字母,|\Sigma| = 26。
方法二:枚举 + 哈希表
思路与算法
在方法一中,我们每次枚举要删除的字符后,都要重新统计所有字符出现的频率,造成了重复的运算。这里我们可以使用哈希表进行优化,在枚举之前,先统计不同字符频率的频率。
假如一个字符出现的频率是 c,那这个字符删除一个后,频率就从 c 变成了 c - 1。我们只需要更新哈希表中 c 和 c - 1 的频率,再判断哈希表的大小是否为一就可以了。如果哈希表大小为 1,则说明剩余每个字母出现频率相同,我们直接返回 true,反之说明删除当前字符不可行,我们把这个字符的频率就从 c - 1 变成 c,更新哈希表进行还原。
要注意更新哈希表后,如果一个键对 \langle \textit{key}, \textit{value} \rangle 中 value} = 0,我们需要手动删除 key。
最后,当我们尝试过所有不同字符后,还没有找到能删除的字符,使得满足要求,我们返回 false。
代码
1 | class Solution { |
1 | class Solution { |
1 | class Solution: |
1 | func equalFrequency(word string) bool { |
1 | public class Solution { |
1 | var equalFrequency = function(word) { |
1 | typedef struct { |
复杂度分析
时间复杂度:O(n+|\Sigma|),其中 n 是字符串 word 的长度, \Sigma 为字符集,在本题中字符集为所有小写字母,|\Sigma| = 26。
空间复杂度:O(|\Sigma|),\Sigma 为字符集,在本题中字符集为所有小写字母,|\Sigma| = 26。