2788-按分隔符拆分字符串
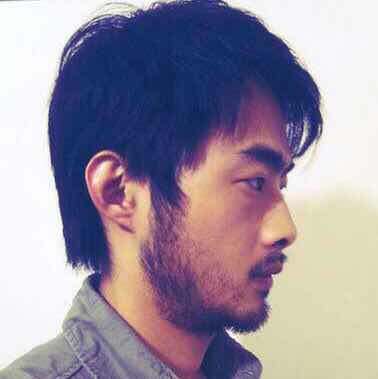
给你一个字符串数组 words
和一个字符 separator
,请你按 separator
拆分 words
中的每个字符串。
返回一个由拆分后的新字符串组成的字符串数组, 不包括空字符串 。
注意
separator
用于决定拆分发生的位置,但它不包含在结果字符串中。- 拆分可能形成两个以上的字符串。
- 结果字符串必须保持初始相同的先后顺序。
示例 1:
**输入:** words = ["one.two.three","four.five","six"], separator = "."
**输出:** ["one","two","three","four","five","six"]
**解释:** 在本示例中,我们进行下述拆分:
"one.two.three" 拆分为 "one", "two", "three"
"four.five" 拆分为 "four", "five"
"six" 拆分为 "six"
因此,结果数组为 ["one","two","three","four","five","six"] 。
示例 2:
**输入:** words = ["easy","problem"], separator = ""
**输出:** ["easy","problem"]
**解释:** 在本示例中,我们进行下述拆分:
"easy" 拆分为 "easy"(不包括空字符串)
"problem" 拆分为 "problem"(不包括空字符串)
因此,结果数组为 ["easy","problem"] 。
示例 3:
**输入:** words = ["|||"], separator = "|"
**输出:** []
**解释:** 在本示例中,"|||" 的拆分结果将只包含一些空字符串,所以我们返回一个空数组 [] 。
提示:
1 <= words.length <= 100
1 <= words[i].length <= 20
words[i]
中的字符要么是小写英文字母,要么就是字符串".,|#@"
中的字符(不包括引号)separator
是字符串".,|#@"
中的某个字符(不包括引号)
355场周赛第一题
周赛时的解题思路
1.split函数 分割。Java 写出来跑的结果不对,怀疑是参数类型问题,就用弱类型的JS来写了
2.示例2、示例3 会有判空问题,用这个 if (sp[j] != “”) res.push(sp[j]); 解决
代码
1 | /** |
周赛后Java解题思路
1.平时写业务代码,Java那遇到分割的情况,也就是按空格或逗号来分割,比如
String[] asp1 = “one two three”.split(“ “); //能出来正常的结果[“one”,”two”,”three”]
String[] asp2 = “one,two,three”.split(“,”); //能出来正常的结果[“one”,”two”,”three”]
都是能正常跑出来的。
2.按照示例1 用点号来分割
String[] asp3 = “one.two.three”.split(“.”); //出来结果居然是个空数组
3.然后评论区告诉我说,Java的split传的参会看作正则,然后我看了下Java的split函数源码上的注释,说是 围绕给定正则表达式的匹配拆分此字符串。
4.然后我去搜了下 asp2、asp3为什么得到的结果不同?得知
5.使用逗号 , 作为分隔符。逗号被视为普通字符而非正则表达式的一部分,因此字符串 “one,two,three” 会按逗号进行拆分
6.使用点号 . 作为分隔符。然而,在正则表达式中,点号 . 有特殊的含义,表示匹配任意字符。因此,这里需要对点号进行转义。由于 Java 字符串中的反斜杠 \ 是一个转义字符,
1 | 所以我们需要使用双反斜杠 \\ 进行转义。因此,正确的语法应该是 split("\\.") |
代码
1 | public List<String> splitWordsBySeparator(List<String> words, char separator) { |