LCP 41-黑白翻转棋
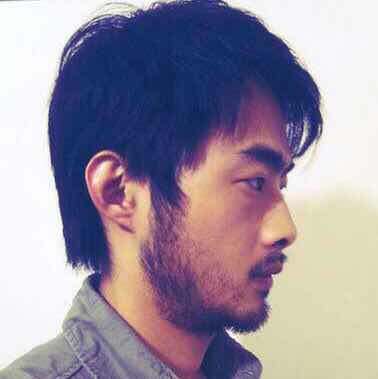
在 n*m
大小的棋盘中,有黑白两种棋子,黑棋记作字母 "X"
, 白棋记作字母 "O"
,空余位置记作"."
。当落下的棋子与其他相同颜色的棋子在行、列或对角线完全包围(中间不存在空白位置)另一种颜色的棋子,则可以翻转这些棋子的颜色。
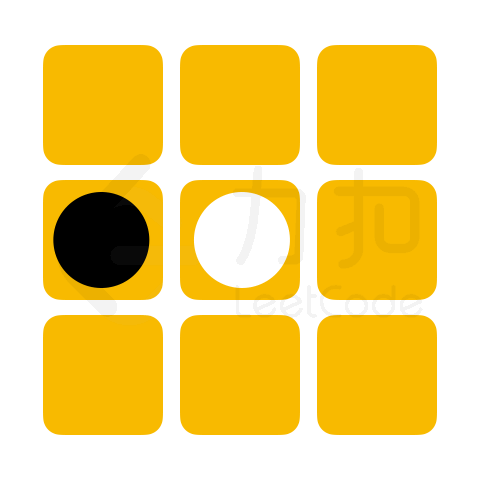{:height=170px}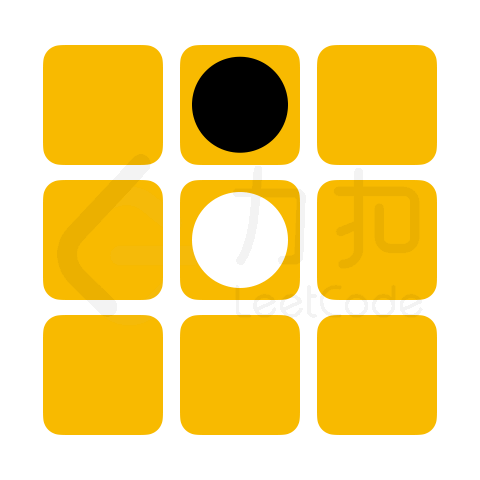{:height=170px}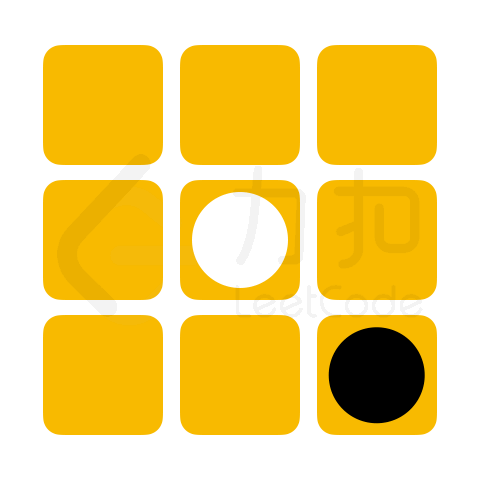{:height=170px}
「力扣挑战赛」黑白翻转棋项目中,将提供给选手一个未形成可翻转棋子的棋盘残局,其状态记作chessboard
。若下一步可放置一枚黑棋,请问选手最多能翻转多少枚白棋。 注意: -
若翻转白棋成黑棋后,棋盘上仍存在可以翻转的白棋,将可以 继续 翻转白棋 - 输入数据保证初始棋盘状态无可以翻转的棋子且存在空余位置 示例
1: > 输入:chessboard = ["....X.","....X.","XOOO..","......","......"]
> >
输出:3
> > 解释: > 可以选择下在 [2,4]
处,能够翻转白方三枚棋子。 示例 2: > 输入:chessboard = [".X.",".O.","XO."]
> > 输出:2
> > 解释: > 可以选择下在 [2,2]
处,能够翻转白方两枚棋子。
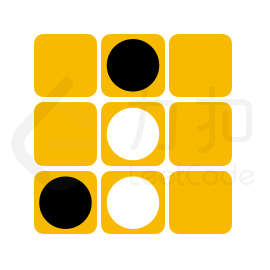 示例 3: >
输入:chessboard = [".......",".......",".......","X......",".O.....","..O....","....OOX"]
> >
输出:4
> > 解释: > 可以选择下在 [6,3]
处,能够翻转白方四枚棋子。
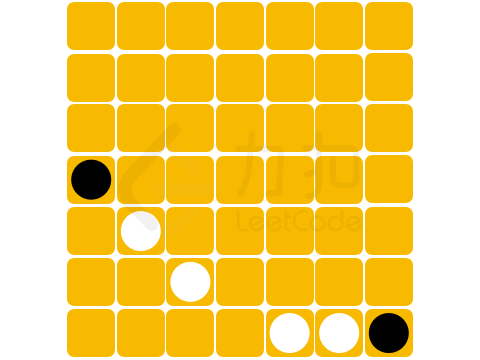 提示: - 1 <= chessboard.length, chessboard[i].length <= 8
- chessboard[i]
仅包含"."、"O"
和 "X"
方法一:广度优先搜索
思路与算法
题目给出一个大小为 n \times m 的黑白棋盘 chessboard,其中黑棋用 X' 表示,白棋用
O’ 表示,空余位置用 `.’ 表示。现在我们可以在棋盘中的一个空余位置下一个黑棋,现在我们称「翻转操作」为:若存在在放置黑棋的位置的行、列或者对角线有另一颗黑棋能完全包围(中间不存在空白位置)白棋,那么我们可以翻转这些白棋,使其变为黑棋,并且这些翻转后得到的新黑棋同样可以进行「翻转操作」。现在我们需要求在放置一次黑棋的情况下最多能翻转多少颗白棋。
我们可以用「广度优先搜索」来解决这个问题,我们对每一个空余位置尝试黑棋放置,用一个队列来存储正在进行「翻转操作」的黑棋位置,若队列非空,我们从队列中取出队首元素,进行行、列和对角线 8 个方向判断是否有可以翻转的白棋——判断沿着方向是否是连续的一段白棋并以另一颗黑棋结尾。若有可以翻转的白棋,则将这些白旗进行翻转,并加入队列中。直至队列为空表示一次放置黑棋结束。
初始可以放置黑棋的全部位置中可以翻转的白棋个数最大值即为最后的答案。
代码
1 | class Solution { |
1 | class Solution { |
1 | public class Solution { |
1 | const int dirs[8][2] = { |
1 | class Solution: |
1 | const dirs = [ |
复杂度分析
- 时间复杂度:O(n^2 \times m^2 \times \max{n, m}),其中 n,m 为给定棋盘的行列数。最多有 n \times m 个初始放置黑棋的位置,每一个位置往 8 个方向进行判断是否能翻转白棋的时间复杂度为 O(\max{n, m}),所以放置初始黑棋后进行「广度优先搜索」的时间复杂度为 O(n \times m \times \max{n, m})。
- 空间复杂度:O(n^2 \times m^2),其中 n,m 为给定棋盘的行列数。主要为每次「广度优先搜索」对初始棋盘进行复制和队列的空间开销。